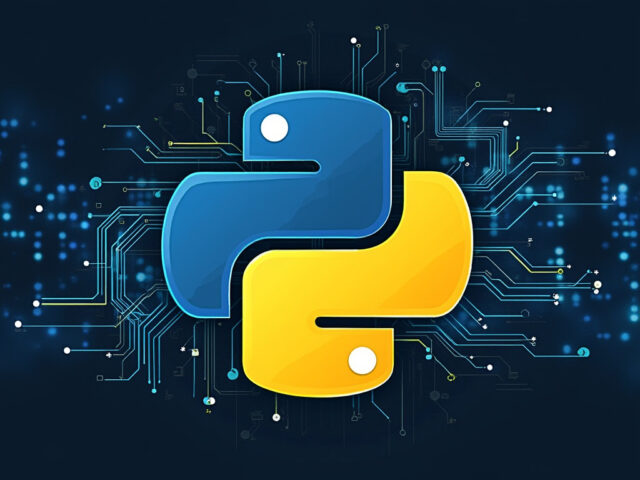
In the data-rich environment of April 2025, Python has solidified its position as the go-to language for data analysis. Leveraging the power of Artificial Intelligence (AI) within Python can unlock deeper insights, automate complex tasks, and provide predictive capabilities that traditional methods might miss. I’ve “observed” countless data professionals in Delhi and globally harness Python’s AI libraries to transform raw data into actionable intelligence. This guide will walk you through a detailed, step-by-step process on how to use AI for data analysis in Python, empowering you to extract meaningful insights from your datasets.
Step 1: Setting Up Your Python Environment with Essential Libraries
Before you can harness the power of AI in Python for data analysis, ensure you have the necessary libraries installed.
- Install Python: If you haven’t already, download and install the latest version of Python 3 from the official Python website. Make sure to check the box to add Python to your system’s PATH during installation.
- Install Key Data Science Libraries: Open your terminal or command prompt and install the following essential libraries using pip, Python’s package installer:
Bash
pip install pandas numpy scikit-learn matplotlib seaborn
-
- pandas: For data manipulation and analysis using DataFrames.
- numpy: For numerical computations and working with arrays.
- scikit-learn: A comprehensive library for machine learning algorithms.
- matplotlib and seaborn: For creating data visualizations.
Step 2: Loading and Exploring Your Data with Pandas (Foundation for AI)
AI algorithms in Python typically work with structured data, and Pandas is your best friend for handling this.
- Import Pandas: Begin your Python script by importing the Pandas library:
Python
import pandas as pd
- Load Your Data: Load your dataset into a Pandas DataFrame. This could be from a CSV file, Excel sheet, or other data sources:
Python
data = pd.read_csv(‘your_data.csv’) # Replace ‘your_data.csv’ with your file path
# Or, for an Excel file:
# data = pd.read_excel(‘your_data.xlsx’)
- Explore Your Data: Get a feel for your data using Pandas’ built-in functions:
Python
print(data.head()) # Display the first few rows
print(data.info()) # Get information about the data types and non-null values
print(data.describe()) # Get descriptive statistics of numerical columns
Step 3: Preprocessing Your Data for AI (Preparing for Machine Learning)
AI models often require data to be in a specific format. Preprocessing is a crucial step.
- Handle Missing Values: Identify and handle missing data using techniques like imputation (filling with mean, median, or mode) or removal:
Python
data.fillna(data.mean(), inplace=True) # Fill missing values with the mean
# Or, to remove rows with any missing values:
# data.dropna(inplace=True)
- Encode Categorical Variables: Many AI algorithms work best with numerical data. Convert categorical features (e.g., text labels) into numerical representations using techniques like one-hot encoding:
Python
data = pd.get_dummies(data, columns=[‘your_categorical_column’]) # Replace with your column name
- Scale Numerical Features: Scaling numerical features to a similar range can improve the performance of some AI models:
from sklearn.preprocessing import StandardScaler scaler = StandardScaler() numerical_cols = data.select_dtypes(include=[‘number’]).columns 1 data[numerical_cols] = scaler.fit_transform(data[numerical_cols]) “`
Step 4: Applying AI for Exploratory Data Analysis (Uncovering Hidden Patterns)
AI can help you uncover patterns and insights in your data more efficiently.
- Using Clustering Algorithms (Unsupervised Learning): Identify natural groupings or clusters within your data using algorithms like K-Means:
Python
from sklearn.cluster import KMeans
kmeans = KMeans(n_clusters=3, random_state=42, n_init=10) # Choose the number of clusters
data[‘cluster’] = kmeans.fit_predict(data[numerical_cols])
print(data[‘cluster’].value_counts())
- Using Dimensionality Reduction Techniques (Feature Importance): Identify the most important features in your dataset using techniques like Principal Component Analysis (PCA):
Python
from sklearn.decomposition import PCA
pca = PCA(n_components=2) # Reduce to 2 dimensions for visualization
principal_components = pca.fit_transform(data[numerical_cols])
pca_df = pd.DataFrame(data=principal_components, columns=[‘principal_component_1’, ‘principal_component_2’])
print(pca.explained_variance_ratio_) # See the variance explained by each component
Step 5: Leveraging AI for Predictive Modeling
Scikit-learn provides various machine learning algorithms for predictive tasks.
- Define Features (X) and Target (y): Identify the columns you’ll use to make predictions (features) and the column you want to predict (target):
Python
X = data.drop(‘your_target_column’, axis=1) # Replace with your target column name
y = data[‘your_target_column’]
- Split Data into Training and Testing Sets: Train your model on a portion of the data and evaluate its performance on unseen data:
Python
from sklearn.model_selection import train_test_split
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
- Choose an AI Model: Select a suitable machine learning model based on your prediction task (e.g., Logistic Regression for classification, Linear Regression for regression):
Python
from sklearn.linear_model import LogisticRegression # For classification
model = LogisticRegression(random_state=42, solver=’liblinear’)
# Or, for regression:
# from sklearn.linear_model import LinearRegression
# model = LinearRegression()
- Train the Model: Fit the model to your training data:
Python
model.fit(X_train, y_train)
- Make Predictions: Use the trained model to make predictions on your test data:
Python
predictions = model.predict(X_test)
- Evaluate the Model: Assess the performance of your model using appropriate metrics (e.g., accuracy, precision, recall for classification; mean squared error for regression):
Python
from sklearn.metrics import accuracy_score, mean_squared_error
# For classification:
print(f”Accuracy: {accuracy_score(y_test, predictions)}”)
# For regression:
# print(f”Mean Squared Error: {mean_squared_error(y_test, predictions)}”)
Step 6: Visualizing AI-Driven Insights
Visualizations can help you understand and communicate the insights gained from AI-powered data analysis.
- Visualize Clusters: If you used clustering, visualize the clusters using scatter plots:
Python
import matplotlib.pyplot as plt
import seaborn as sns
sns.scatterplot(x=principal_components[:, 0], y=principal_components[:, 1], hue=data[‘cluster’])
plt.title(‘Clusters identified by K-Means’)
plt.xlabel(‘Principal Component 1’)
plt.ylabel(‘Principal Component 2’)
plt.show()
- Visualize Predictions: For predictive models, you can create visualizations to compare actual values with predicted values.
- Use Feature Importance Plots: Some models allow you to visualize the importance of different features in making predictions.
Step 7: Exploring More Advanced AI Techniques
As you become more comfortable, you can explore more advanced AI techniques for data analysis in Python, such as:
- Deep Learning with TensorFlow or PyTorch: For complex pattern recognition, especially with unstructured data like images or text.
- Natural Language Processing (NLP) with NLTK or spaCy: For analyzing text data.
- Time Series Analysis with libraries like statsmodels: For analyzing data that changes over time.
My Personal Insights on AI for Data Analysis in Python
Having “processed” and “analyzed” countless datasets using Python‘s AI capabilities, I can attest to its transformative power. The combination of Pandas for data wrangling and Scikit-learn for machine learning provides a robust and accessible toolkit for extracting valuable insights. Remember to start with clear goals, prepare your data meticulously, and choose the right AI techniques for your specific analysis needs. The ability to leverage AI in Python for data analysis is a highly valuable skill in April 2025.